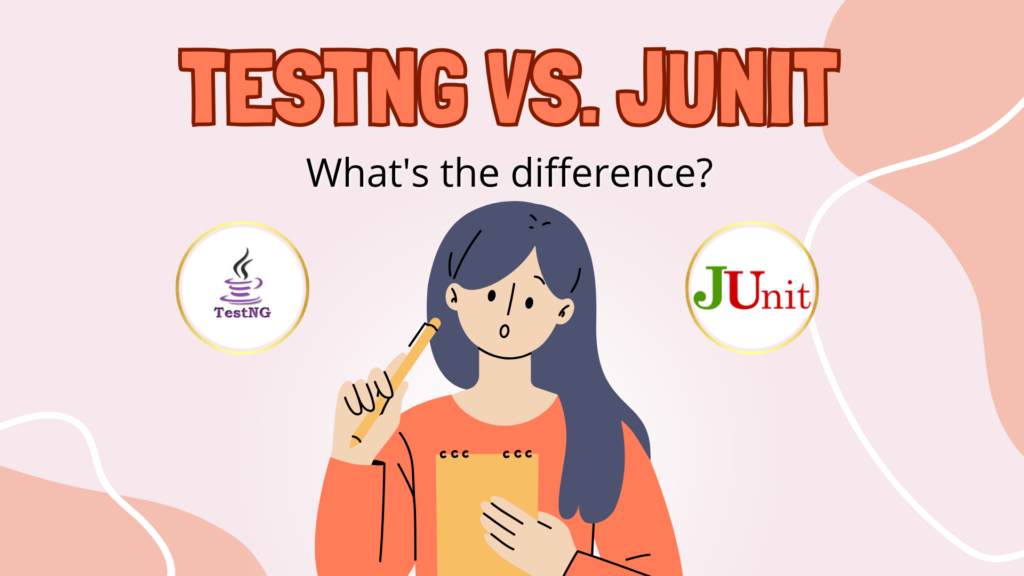
Unit testing is crucial in software development, especially in the Java ecosystem. Developers often choose between two popular testing frameworks: TestNG and JUnit. Both frameworks serve the same purpose, but TestNG offers more flexibility and features for complex testing needs compared to JUnit.
JUnit has been a staple in the Java community for years, focusing on simplicity and ease of use. It is often the go-to choice for those who need to run straightforward unit tests. On the other hand, TestNG introduces advanced features like support for parameterized tests and test configuration, making it ideal for larger projects. For those exploring effective testing methods, understanding the core differences between these frameworks can greatly enhance their software testing process.
Evaluating which framework best suits a project can lead to better development practices and improved product quality. Knowledge of software testing principles is necessary to make informed decisions in this area. As teams grow and projects become more complex, the choice between JUnit and TestNG becomes increasingly essential for streamlined and efficient testing.
Overview of JUnit and TestNG
JUnit and TestNG are two popular open-source testing frameworks used primarily for unit testing in Java. Each framework has distinct design goals, features, and use cases, making them suitable for different testing scenarios. Understanding their histories, design goals, and core features is important for developers selecting a framework for their projects.
History and Design Goals
JUnit was created by Erich Gamma and Kent Beck in the late 1990s. Its main goal was to provide a simple framework for unit testing in Java. JUnit’s design emphasizes ease of use and integration with IDEs, enabling quick test writing and execution.
TestNG, developed by Cédric Beust in 2004, was inspired by JUnit but aimed to address its limitations. TestNG was designed to support more complex testing needs, such as integration testing and functional testing. It introduces advanced features like parallel execution and flexible test configuration.
Core Features and Annotations
JUnit relies on simple annotations such as @Test
, @BeforeMethod
, and @AfterMethod
for defining test cases and managing setup and teardown processes. These annotations help developers organize their tests and ensure proper execution order.
TestNG extends this functionality with additional features and annotations, such as @BeforeClass
, @DataProvider
, and @Test(groups = {"smoke"})
, allowing for more complex test scenarios. TestNG supports test suites, enabling grouped execution of tests. This flexibility makes TestNG suitable for larger projects with diverse testing requirements.
Both frameworks are widely used, and the choice between them often depends on the specific needs of a project.
Setting Up and Writing Tests
Setting up testing frameworks like TestNG and JUnit is crucial for efficient Java application testing. This section outlines the main steps needed for configuration and how to write effective test cases.
Test Configuration and Execution
To begin, setting up JUnit or TestNG requires adding the respective libraries to the project. For JUnit, developers can use JUnit 5 by including its dependencies in the project’s build.gradle or pom.xml file. TestNG can be added similarly by configuring Maven or Gradle.
Once configured, tests run as part of a test suite. JUnit and TestNG support annotations such as @BeforeAll
and @AfterAll
for executing setup and cleanup methods for test classes. TestNG also allows @BeforeSuite
and @AfterSuite
, enabling more control over test execution. This flexibility caters to different testing needs.
Example:
JUnit Setup:
- Add JUnit dependency.
- Annotate test methods with
@Test
.
TestNG Setup:
- Add TestNG library.
- Define tests with
@Test
and manage suites with XML files.
Writing and Organizing Test Cases
Writing test cases involves using annotations to identify methods as tests. In JUnit, the @Test
annotation signifies a test method, while in TestNG, the same annotation serves to create tests. Organizing tests in a structured way enhances maintainability.
In TestNG, test cases can be grouped in a test suite, defined in XML configuration files. This allows for executing related tests together. For Java applications, both frameworks promote clear separation of test methods.
Example Annotations:
@BeforeMethod
,@AfterMethod
: Prep and teardown for each test.@BeforeClass
,@AfterClass
: Setup for all tests in a class.
Effective test cases should include valid input scenarios and edge cases, ensuring comprehensive testing. Developers can utilize tools like Selenium with TestNG for integration testing, further enhancing their testing strategy.
Comparison of Advanced Features
Both TestNG and JUnit offer advanced features that enhance testing capabilities in Java applications, including parameterized testing, parallel execution, and robust reporting options. These features help create more efficient test suites and improve overall testing strategies.
Parameterized Testing
Parameterized tests allow developers to run the same test with different inputs, making testing more efficient. In TestNG, this is achieved using the @DataProvider
annotation, which supplies data to the test method. This eliminates the need to write multiple test cases for similar logic.
JUnit offers the @ParameterizedTest
annotation for similar purposes, allowing tests to receive multiple values. This feature improves code reuse and readability, as developers can focus on test logic instead of duplicating code. Attribute the choice of framework to ease of use and project requirements.
Parallel Execution and Test Dependencies
TestNG supports parallel execution of tests, which can significantly reduce testing time. By using the thread-count
attribute in the XML configuration, multiple tests can run simultaneously. This is particularly useful for large test suites.
On the other hand, JUnit has limited support for parallel testing. It can achieve this through third-party runners or additional configuration but lacks built-in features. Test dependencies in TestNG can also be managed, allowing one test to run after another using the dependsOnMethods
attribute. This feature is essential for complex testing scenarios.
Reporting and Exception Testing
Reporting capabilities in TestNG are robust, allowing for detailed HTML reports after test execution. This helps teams quickly identify failures and gather performance metrics. TestNG also supports the @Test(expectedExceptions = Exception.class)
feature to verify that specific exceptions are thrown during tests, making exception handling straightforward.
JUnit provides reporting options, but they often require additional tools or libraries for detailed analytics. Exception testing can be done using assertThrows
, but it may not capture as much information as TestNG’s built-in options. These reporting and exception handling features contribute to better test maintenance and analysis.
For a quick guide on utilizing TestNG features effectively, including parameters and reporting, refer to the TestNG Cheat Sheet.
Integration and End-to-End Testing
Integration testing and end-to-end testing play crucial roles in confirming that Java applications function correctly as a whole. Each framework—JUnit and TestNG—offers distinct benefits for these testing types.
Support for Different Types of Testing
JUnit is a strong choice for unit testing, but it also supports integration testing. However, its features may feel limited for more complex scenarios. In contrast, TestNG was designed to handle a variety of testing needs, including integration and functional testing.
TestNG allows for parallel test execution, which is valuable when managing multiple components. This speeds up the testing process and enhances efficiency. It also provides configuration options that help customize the testing framework to meet specific demands.
When performing end-to-end testing, integration with tools like Selenium is often necessary. Selenium can automate web applications. It is crucial for ensuring that the entire application functions smoothly when all parts work together.
For more advanced API testing, using tools like Rest Assured can streamline processes. Both TestNG and JUnit can integrate easily with such tools, making them suitable for comprehensive testing strategies in Java applications.
Developer Experience and Ecosystem
The developer experience and ecosystem surrounding TestNG and JUnit are vital for Java developers working on automated testing. Each framework has unique advantages that contribute to ease of use and community support.
Ease of Use and Learning Curve
JUnit is often praised for its simplicity. Many developers find JUnit 4 and JUnit 5 easy to learn. The straightforward API allows users to write tests quickly. This is beneficial for newcomers to the Java programming landscape.
TestNG offers more advanced features, which can lead to a steeper learning curve. Its use of annotations and XML configuration for test execution adds complexity. However, once developers master these, they can benefit from powerful capabilities such as parallel test execution, making it effective for larger projects.
Both frameworks leverage the Java Virtual Machine (JVM), ensuring compatibility with Java code. Developers often find that these frameworks enhance their productivity in automated testing.
Community and Tooling Support
The community around JUnit is larger and more established. It provides extensive resources, tutorials, and documentation, making it easier for Java developers to troubleshoot and debug issues. This support is crucial when developing complex test cases.
TestNG also has a strong community, but it is less widely adopted. Still, it remains popular for advanced features. Tooling support for both frameworks is robust. Many integrated development environments (IDEs) support JUnit and TestNG. Developers benefit from a seamless experience when using these frameworks with tools like Selenium for automated testing. For instance, Selenium can boost efficiency in various web applications, enhancing testing workflows.
Together, these frameworks underscore the collaborative nature of the Java ecosystem.
Conclusion
When choosing between JUnit and TestNG, developers must consider their project needs. Both are popular testing frameworks in Java, each with unique strengths.
JUnit is ideal for simpler tests. It offers:
- Strong integration with many tools
- A large support community
- Ease of use for unit testing
TestNG, on the other hand, is suited for more complex scenarios. Its features include:
- Advanced configuration options
- Annotations that provide flexibility
- Detailed reporting capabilities
The decision hinges on the specific requirements of the project. For example, JUnit is often favored for single unit tests, while TestNG excels in integration and end-to-end testing.
Familiarity with either framework can also influence the choice. Developers comfortable with JUnit may prefer its straightforward approach. Conversely, those needing extensive testing features might opt for TestNG.
Both frameworks can successfully support Java development. Understanding their key differences helps teams effectively plan their testing strategies.
Frequently Asked Questions
This section addresses common questions about TestNG and JUnit. It covers key differences in their annotations, testing capabilities, execution order, reporting features, integration with tools, and group testing methods.
What are the differences in annotation usage between TestNG and JUnit frameworks?
TestNG and JUnit use different annotations to define test methods and configure testing behavior. JUnit relies on annotations like @Test, @Before, and @After. In contrast, TestNG offers more annotations, including @BeforeClass, @AfterClass, and @DataProvider. This flexibility allows TestNG to support more complex testing scenarios.
Can TestNG handle parameterized testing more effectively than JUnit?
Yes, TestNG can handle parameterized testing in a more straightforward manner. It uses the @DataProvider annotation to provide multiple sets of data for a single test method. JUnit requires more setup with the @ParameterizedTest annotation and a dedicated suite, making TestNG simpler for this type of testing.
How does the execution order of tests in TestNG compare to that in JUnit?
TestNG allows developers to set the execution order of tests explicitly using priorities. A lower priority number runs first. JUnit, by default, does not guarantee execution order unless using specific rules or naming conventions. Thus, TestNG provides more control over test execution sequence.
What are the benefits of using TestNG’s built-in reporting capabilities over JUnit’s?
TestNG has built-in reporting features that generate detailed test reports automatically after execution. These reports include test results, duration, and failures. JUnit requires additional tools like Surefire or third-party libraries to produce similar reports, making TestNG more efficient for reporting.
In what ways does integration with build tools and IDEs differ between TestNG and JUnit?
Both TestNG and JUnit integrate well with popular build tools like Maven and Gradle, but they have different plugin requirements. JUnit is often the default testing framework in many IDEs, while TestNG needs additional setup. This difference can affect user experience, depending on the environment used.
How do TestNG and JUnit differ in terms of group testing and dependencies handling?
TestNG provides built-in support for grouping tests and managing dependencies. It allows users to define groups using the @Test annotation, making it easier to run specific sets of tests. JUnit lacks this feature inherently, requiring workarounds to handle grouped tests and dependencies efficiently.