Page Object Model (POM) is a design pattern used in Selenium WebDriver to make test scripts more readable and easy to maintain. It helps organize code by creating separate classes for each web page or component. This approach reduces duplication and improves test case management.
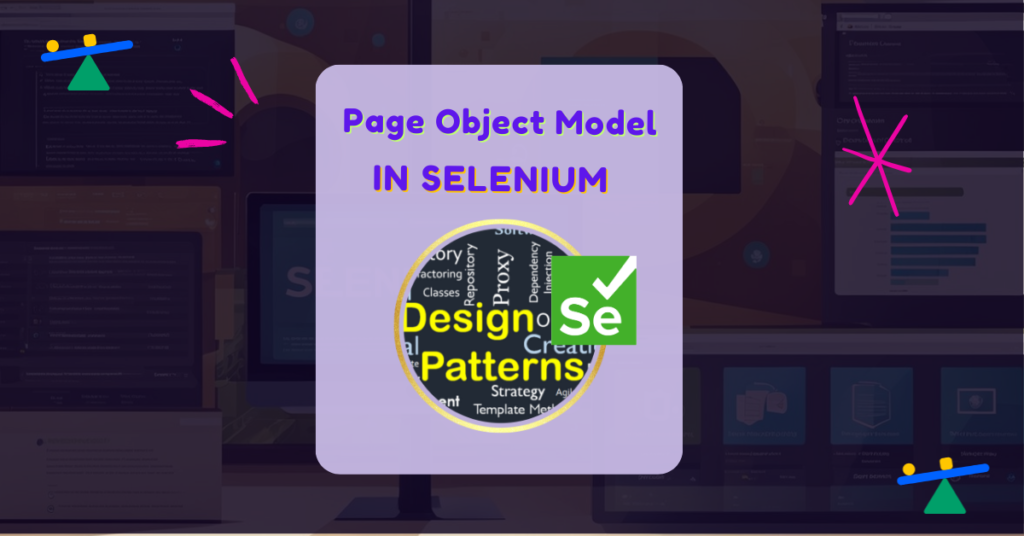
The Page Object Model creates an object repository for storing web elements, which allows testers to write cleaner and more efficient code. By separating page elements from test methods, POM makes it easier to update tests when changes occur in the application’s user interface. This separation also enhances code reusability across different test cases.
Using POM in Selenium improves test maintenance and reduces the chances of errors in test scripts. It allows testers to create more robust automation frameworks that can handle complex web applications. The Page Object Model also makes it easier for teams to collaborate on test automation projects, as the code structure is more organized and intuitive.
Key Takeaways
- POM separates page elements from test methods for better code organization
- It reduces code duplication and improves test script maintenance
- POM enhances collaboration and scalability in test automation projects
Understanding Page Object Model (POM)
Page Object Model is a design pattern that improves test automation in Selenium. It creates separate objects for each webpage, making tests more organized and easier to maintain.
Conceptual Overview
Page Object Model (POM) is a design pattern for test automation. It creates a layer between test scripts and the user interface. In POM, each web page is a separate class file. These class files contain the page’s elements and methods to interact with them.
POM separates the test code from the page-specific code. This separation makes tests cleaner and more robust. When a page’s layout changes, only the page object needs updating, not the tests.
The pattern follows object-oriented principles. It encapsulates webpage details and provides an interface for tests to use.
Components of POM
Page classes are the main components of POM. Each class represents a single web page or component. These classes contain:
- Web elements: Buttons, text fields, and other UI items
- Locators: Ways to find elements on the page
- Page methods: Functions to interact with the page
Web elements are defined using locators like ID, name, or XPath. Page methods perform actions like clicking buttons or entering text. They often return other page objects, matching the flow of the application.
A typical page class might look like this:
public class LoginPage {
WebElement username = driver.findElement(By.id("user"));
WebElement password = driver.findElement(By.id("pass"));
WebElement loginButton = driver.findElement(By.id("login"));
public void login(String user, String pass) {
username.sendKeys(user);
password.sendKeys(pass);
loginButton.click();
}
}
This structure keeps page-specific code separate from test logic.
Benefits of Implementing POM
POM offers several advantages for Selenium test automation:
- Improved maintainability: Changes to the UI only require updates in one place.
- Increased readability: Tests become more descriptive and easier to understand.
- Reduced code duplication: Common page actions are defined once and reused.
POM makes tests more stable. They’re less likely to break when small UI changes occur. This stability saves time on test maintenance.
The pattern also supports better collaboration. Testers can work on different page objects without conflicts. Developers can easily understand and contribute to the test code.
Reusability is a key benefit. Page objects can be shared across multiple test cases. This sharing reduces the overall amount of code needed.
Setting Up Selenium WebDriver with POM
Setting up Selenium WebDriver with Page Object Model (POM) involves configuring the WebDriver and integrating it with the POM structure. This approach enhances test script organization and maintainability.
WebDriver Basics
Selenium WebDriver is a tool for automating web browser interactions. To set it up, first install the Selenium package and the appropriate browser driver.
Here’s a basic setup for Chrome:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://www.example.com")
This code launches Chrome and navigates to the specified URL. WebDriver provides methods to interact with web elements, such as:
find_element()
: Locates a single elementclick()
: Clicks on an elementsend_keys()
: Enters text into a field
These methods form the foundation for interacting with web applications in automated tests.
Integrating POM with WebDriver
POM integration with WebDriver involves creating separate classes for each page in the web application. Each class contains methods that represent actions on that page.
A typical page class structure looks like this:
from selenium.webdriver.common.by import By
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, "username")
self.password_field = (By.ID, "password")
self.login_button = (By.ID, "login")
def enter_username(self, username):
self.driver.find_element(*self.username_field).send_keys(username)
def enter_password(self, password):
self.driver.find_element(*self.password_field).send_keys(password)
def click_login(self):
self.driver.find_element(*self.login_button).click()
This approach separates page-specific code from test logic. It makes tests more readable and easier to maintain. Page Factory, an improvement to POM, uses annotations like @FindBy
to locate elements. This further simplifies element management in page classes.
Designing Page Objects
Designing page objects involves creating a structured representation of web pages for efficient test automation. This approach enhances code organization and simplifies maintenance of Selenium scripts.
Page Classes Structure
Page classes form the backbone of the Page Object Model. Each web page is represented by a separate class. These classes contain methods that perform actions on the page and elements that represent the UI components.
A typical page class includes:
- Private variables for web elements
- Public methods for page interactions
- Constructors to initialize the page
Example structure:
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = driver.find_element(By.ID, "username")
self.password_field = driver.find_element(By.ID, "password")
self.login_button = driver.find_element(By.XPATH, "//button[@type='submit']")
def enter_username(self, username):
self.username_field.send_keys(username)
def enter_password(self, password):
self.password_field.send_keys(password)
def click_login(self):
self.login_button.click()
This structure improves readability and makes the code more maintainable.
Handling Web Elements
Web elements are the building blocks of page objects. They represent UI components like buttons, input fields, and dropdowns. Proper handling of these elements is crucial for robust automation.
Key points for handling web elements:
- Use descriptive names for element variables
- Implement reliable locator strategies (ID, name, CSS, XPath)
- Group related elements together
Example of handling web elements:
class SearchPage:
def __init__(self, driver):
self.search_input = driver.find_element(By.ID, "search-box")
self.search_button = driver.find_element(By.XPATH, "//button[text()='Search']")
self.result_list = driver.find_elements(By.CLASS_NAME, "result-item")
def perform_search(self, query):
self.search_input.clear()
self.search_input.send_keys(query)
self.search_button.click()
def get_result_count(self):
return len(self.result_list)
This approach creates an object repository for web elements, making it easier to update and maintain the automation code.
Writing Test Cases Using POM
The Page Object Model enhances test case creation in Selenium. It boosts code reusability and makes tests easier to update. Let’s explore how to structure test scripts and use testing frameworks with POM.
Structuring Test Scripts
POM simplifies test script structure. Each web page gets its own class file. These files hold the page’s elements and actions. Test scripts then use these page objects.
A typical test script with POM looks like this:
- Create page object instances
- Perform actions using page methods
- Make assertions to check results
For example, a login test might do this:
LoginPage loginPage = new LoginPage(driver);
HomePage homePage = loginPage.login("user", "pass");
assertTrue(homePage.isLoggedIn());
This setup keeps page details separate from test logic. It makes scripts cleaner and easier to maintain.
Leveraging Testing Frameworks
Testing frameworks like JUnit, TestNG, and PyTest work well with POM. They add helpful features to Selenium tests.
JUnit and TestNG offer annotations for test setup and teardown. This helps manage test environments. PyTest uses fixtures for similar purposes.
These frameworks also provide powerful assertion methods. They make it easy to check test results.
Here’s a simple TestNG example:
@Test
public void loginTest() {
LoginPage loginPage = new LoginPage(driver);
HomePage homePage = loginPage.login("user", "pass");
Assert.assertTrue(homePage.isLoggedIn());
}
Using these frameworks with POM creates robust, maintainable test suites. They help organize tests and report results clearly.
Maintaining and Scaling POM
The Page Object Model (POM) helps keep Selenium tests organized as projects grow. It makes updating and expanding test suites easier over time.
Adapting to UI Changes
When web pages change, POM allows quick updates. Testers only need to modify the relevant page object, not every test script. This saves time and reduces errors.
UI elements are defined in one place. If a button moves or changes its ID, only that definition needs updating. All tests using that element will work without changes.
POM also helps handle dynamic content. Page objects can include methods to wait for elements to load. This makes tests more stable when dealing with slow-loading pages or AJAX updates.
Enhancing Code Maintainability
POM improves test code quality. It separates page structure from test logic. This makes scripts easier to read and update.
Each page object acts as a single source of truth for a web page. Developers can quickly find and fix issues related to specific pages.
Reusable components are a key benefit. Common actions like logging in can be defined once and used in many tests. This cuts down on duplicate code.
POM also supports better naming conventions. Methods in page objects can use clear, descriptive names. This makes test scripts more understandable, even for non-technical team members.
Advanced POM Strategies
The Page Object Model can be enhanced with advanced techniques to handle complex web elements and dynamic content. These strategies improve test reliability and maintainability.
Implementing Page Factories
Page Factory is a built-in feature in Selenium that simplifies element initialization. It uses the @FindBy annotation to locate web elements. This annotation supports various locator strategies like ID, name, and CSS selectors.
To use Page Factory, developers call the InitElements method. This method creates the web elements defined in the page object. Here’s an example:
public class LoginPage {
@FindBy(id = "username")
private WebElement usernameField;
@FindBy(name = "password")
private WebElement passwordField;
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
}
}
Page Factory also allows for lazy loading of elements. This means elements are only located when they’re needed, improving test performance.
Dynamic Elements and Ajax Handling
Modern web apps often use Ajax to load content dynamically. This can cause issues with element location. The AjaxElementLocatorFactory helps manage this challenge.
This factory waits for elements to be present before interacting with them. It’s used like this:
PageFactory.initElements(new AjaxElementLocatorFactory(driver, 30), this);
For highly dynamic pages, JavaScript can be used to locate elements. Selenium’s JavascriptExecutor allows running JS code to find or interact with elements.
When dealing with complex Ajax scenarios, Selenium Grid can be helpful. It allows running tests in parallel, reducing overall test execution time.
Adopting POM with Various Programming Languages
The Page Object Model can be implemented in different programming languages used for Selenium automation. Java and Python are two popular choices for creating POM frameworks, each with their own specific tools and approaches.
POM with Java
Java provides robust support for implementing POM in Selenium. The Page Factory class is a key component in Java-based POM frameworks. It uses annotations to initialize web elements.
To set up a POM in Java:
- Create a class for each web page
- Use @FindBy annotations to locate elements
- Initialize elements with PageFactory.initElements() method
Here’s a simple example:
public class LoginPage {
@FindBy(id = "username")
private WebElement usernameField;
@FindBy(id = "password")
private WebElement passwordField;
public LoginPage(WebDriver driver) {
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
}
}
JUnit is often used with Java POM for test execution and assertions. This combination allows for clean, maintainable test code.
POM with Python
Python offers a straightforward approach to implementing POM in Selenium. While it doesn’t have a built-in Page Factory like Java, Python’s simplicity allows for easy creation of page objects.
To implement POM in Python:
- Create a class for each page
- Define methods to interact with page elements
- Use locators to find elements
Here’s a basic example:
from selenium.webdriver.common.by import By
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, "username")
self.password_field = (By.ID, "password")
def login(self, username, password):
self.driver.find_element(*self.username_field).send_keys(username)
self.driver.find_element(*self.password_field).send_keys(password)
PyTest is a popular testing framework used with Python POM implementations. It provides features for test organization and execution.
Real-World Applications and Case Studies
Page Object Model in Selenium has proven effective for many companies in automating web testing. It helps organize code and make tests easier to maintain. Let’s look at a real example to see how it works in practice.
Example Project Analyses
A large e-commerce company used Page Object Model to improve their Selenium automation for testing their online store. They created separate classes for the HomePage, ProductPage, and CheckoutPage.
The HomePage class contained methods for searching products and navigating menus. The ProductPage class handled adding items to the cart. The CheckoutPage class managed entering payment details and completing orders.
This structure made their tests clearer and easier to update. When the website design changed, they only needed to update the relevant page object, not every test.
Another case study involves a banking app. The team used Page Object Model to test login, account views, and transfers. They found it easier to add new tests as features were developed.
These examples show how Page Object Model can boost efficiency in test automation for complex web applications. It helps teams write more stable and maintainable test code.
Frequently Asked Questions
The Page Object Model offers key benefits for Selenium test automation. It improves code reusability, maintainability, and readability. Here are answers to common questions about implementing and using POM effectively.
How do you implement the Page Object Model in Selenium with Python?
To implement POM in Selenium with Python:
- Create a separate class for each web page.
- Define methods in each class to interact with page elements.
- Use the @FindBy annotation to locate elements.
Example:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = (By.ID, "username")
self.password_field = (By.ID, "password")
self.login_button = (By.ID, "login")
def enter_username(self, username):
WebDriverWait(self.driver, 10).until(EC.presence_of_element_located(self.username_field)).send_keys(username)
def enter_password(self, password):
self.driver.find_element(*self.password_field).send_keys(password)
def click_login(self):
self.driver.find_element(*self.login_button).click()
This approach keeps page objects focused on a single page’s functionality.
Can you provide an example of the Page Object Model framework in Java using Selenium?
Here’s a basic example of POM in Java with Selenium:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.PageFactory;
public class LoginPage {
WebDriver driver;
@FindBy(id = "username")
WebElement usernameField;
@FindBy(id = "password")
WebElement passwordField;
@FindBy(id = "login")
WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void enterUsername(String username) {
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
passwordField.sendKeys(password);
}
public void clickLogin() {
loginButton.click();
}
}
This example uses the @FindBy annotation to locate elements on the page.
What is the architecture of the Page Object Model framework in Selenium?
The POM architecture in Selenium consists of:
- Page Objects: Classes representing web pages with methods for interactions.
- Test Classes: Contains test scripts using page objects.
- Base Class: Handles common functions like driver initialization.
This structure separates test logic from page-specific code, making tests more maintainable.
How does the Page Object Model differ from Page Factory in Selenium?
POM and Page Factory are related but different concepts:
- POM is a design pattern for creating object repositories for web UI elements.
- Page Factory is a built-in way in Selenium to implement POM.
Page Factory uses the @FindBy annotation to locate elements, while basic POM uses methods like driver.findElement().
What are the advantages of using the Page Object Model in Selenium testing?
POM offers several benefits for Selenium testing:
- Improved code reusability
- Enhanced test maintenance
- Reduced code duplication
- Better readability
These advantages make tests more modular and easier to update when the UI changes.
How do you integrate the Page Object Model with Playwright for end-to-end testing?
To use POM with Playwright:
- Create page object classes for each page.
- Use Playwright’s locator methods in page objects.
- Implement methods for page interactions.
Example:
from playwright.sync_api import Page
class LoginPage:
def __init__(self, page: Page):
self.page = page
self.username_input = page.locator("#username")
self.password_input = page.locator("#password")
self.login_button = page.locator("#login")
def login(self, username: str, password: str):
self.username_input.fill(username)
self.password_input.fill(password)
self.login_button.click()
This approach applies POM principles to Playwright, improving test organization and maintenance.
Is there a recommended course for learning Selenium with implementation of Page Object Model from scratch?
For anyone looking to master Selenium with TestNG, consider enrolling in the Selenium Automation Testing for Beginners Course on Udemy. This course covers essential concepts and advanced techniques, helping learners effectively use Selenium in real-world scenarios.