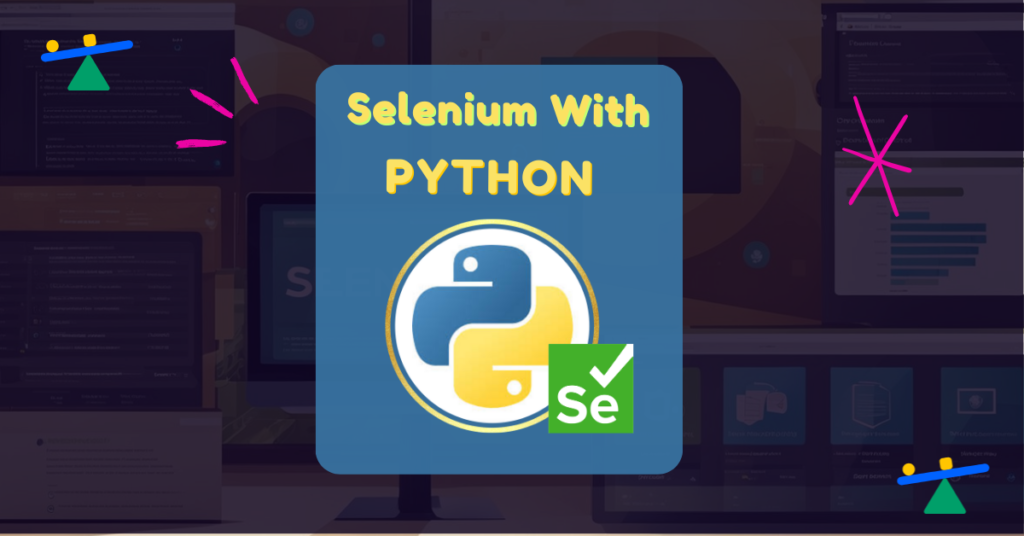
Selenium with Python is an essential tool for anyone looking to automate web tasks efficiently. It provides a powerful open-source framework that allows users to control web browsers programmatically. With Python’s simple syntax and Selenium’s robust functionalities, creating automated tests or scraping data from websites becomes more accessible and efficient.
Setting up the Selenium environment and mastering WebDriver are crucial steps for effective automation. Users can enhance their browser interactions and implement synchronization strategies to ensure reliable automated testing. This combination not only improves workflow but also helps uncover potential issues faster, ultimately making software development more effective.
Selenium’s versatility extends to advanced testing techniques and framework integration, making it a preferred choice in various industries. Learning the fundamentals of web automation opens new opportunities for developers, testers, and anyone interested in improving their productivity through automation.
Key Takeaways
- Selenium with Python simplifies web automation tasks.
- Mastering WebDriver improves testing effectiveness.
- Advanced techniques enhance the automation experience.
Setting Up the Selenium Environment
Setting up the Selenium environment is crucial for running automated tests effectively. This includes installing the necessary libraries, configuring browsers, and understanding how to use WebDriver executables to control browser actions.
Installation of Selenium and WebDrivers
To begin using Selenium, it is important to install the Selenium package. This can be done easily using the command line. The command pip install selenium
allows users to install the latest version available.
After installing Selenium, the next step is to set up the WebDriver for the browser of choice. For instance, Chrome requires the ChromeDriver, Firefox uses GeckoDriver, and Edge has its own WebDriver. Each of these can be downloaded from their respective official websites. For a seamless experience, ensure that these drivers are compatible with the browser version being used.
Configuring Browsers for Selenium
Once the necessary drivers are installed, configuring the browsers properly is essential. Each browser requires specific settings for Selenium to control it. For Chrome and Firefox, the path to the WebDriver must be set in the system’s environment variables.
In addition to standard configurations, users can set their browsers to run in headless mode. This allows for running tests without opening a visible browser window, which is useful for efficiency. By adding certain options in the Selenium code, such as options.add_argument("--headless")
for Chrome, users can execute tests quietly in the background.
Understanding WebDriver Executables
WebDriver executables act as a bridge between your Selenium scripts and the browser. These executables are responsible for driving the browser through the Selenium API. It is crucial to have the executable files for the specific browsers installed and accessible.
When configuring the Selenium environment, users must ensure the WebDriver executable is in their system’s PATH or specify its location directly in the code. For instance, if using GeckoDriver, the path may look like this:
driver = webdriver.Firefox(executable_path='/path/to/geckodriver')
Understanding this linking mechanism is vital for successful automation. By following these steps, users can start automating web interactions effectively. To learn more about getting started with Selenium, one can consult resources available for beginners.
Before we proceed further, I would like to share our exclusive Selenium with Python Cheat sheet as one of the take away from this article. You can also get free PDF version from this link.
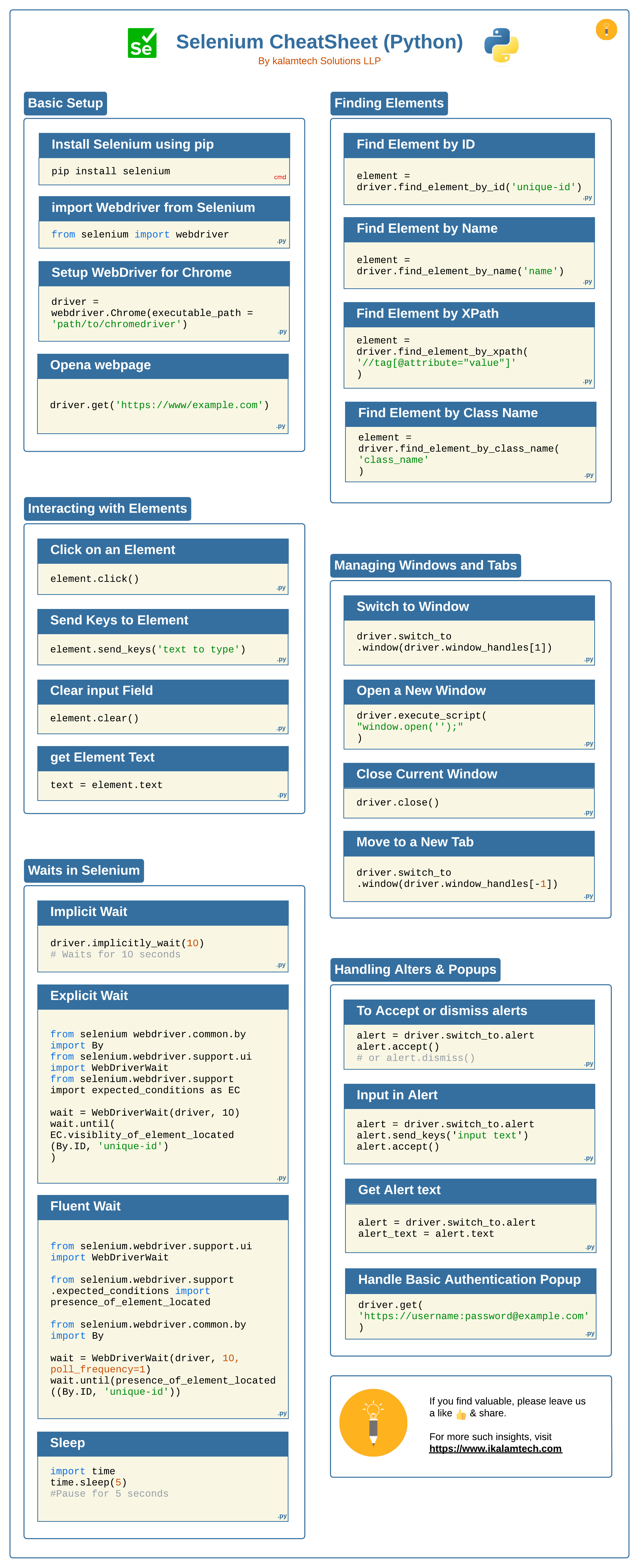
Fundamentals of Web Automation with Selenium
Web automation involves using tools to perform tasks on a web browser automatically. Selenium is a popular framework that makes this process easier, allowing developers to write scripts that simulate user interactions with web elements like buttons and input fields.
Basic Web Automation Tasks
Basic tasks in web automation include navigation, filling out forms, and clicking buttons. Selenium scripts automate these actions by controlling the browser. For example, a script can open a webpage, enter text into input fields, and submit a form.
Using find_element
, users can locate specific elements on a webpage. They can identify elements by their ID, name, class, or CSS selectors. This flexibility allows for precise interactions where scripts can simulate real user behavior efficiently.
Interacting with Web Elements
Selenium enables interaction with various web elements such as buttons, input fields, and dropdowns. To interact with a button, the script can use the click()
method. For input fields, the send_keys()
function allows text entry. This means users can enter data into forms automatically.
Dropdowns require different methods for interaction. Selenium provides the Select
class to manage these elements easily. It allows selection by visible text or index, ensuring that the right options are always chosen during automation.
Selenium Commands and Operations
Selenium offers a variety of commands to help automate web tasks effectively. These include actions like get()
, which opens a URL, and quit()
, which closes the browser after tasks are completed.
Moreover, using the Python bindings, developers can write concise and readable scripts. A comprehensive understanding of these commands enhances a developer’s ability to create robust test automation scripts. Resources like the Selenium Cheat Sheet provide essential commands to streamline this process.
By mastering these fundamental aspects, developers can harness the full power of Selenium for effective web automation.
Locator Strategies and Techniques
Locator strategies are essential for interacting with elements on a web page using Selenium. These strategies help identify elements uniquely, allowing for effective automation. Various methods such as ID, name, class, and XPath are available for choosing the best approach.
Finding Elements Using Selenium
Finding elements is the first step in web automation. Selenium provides multiple methods for locating elements.
find_element_by_id: This method locates an element using its unique ID. It is often the fastest and most reliable choice.
find_element_by_name: This method targets elements based on the
name
attribute. It works well when IDs are not available.find_element_by_class_name: This option identifies elements using their class attribute. It is useful when working with multiple similar elements.
find_element_by_tag_name: This method allows users to find elements by their HTML tag, which can be helpful for selecting specific types of elements like buttons or links.
Advanced Locator Methods
Advanced locator techniques provide more flexibility in identifying elements.
find_element_by_xpath: This powerful method allows users to navigate the document structure to find elements. It can target elements based on conditions or relationships in the XML tree.
find_element_by_link_text: This option is specifically for finding links by their visible text. It is perfect for web pages with many hyperlinks.
find_element_by_css_selector: This modern approach utilizes CSS selectors to locate elements. It offers greater precision and can combine multiple locator strategies.
Using these advanced methods enhances the ability to interact with dynamic and complex web pages effectively.
Mastering Selenium WebDriver
Selenium WebDriver is essential for automating web applications. Understanding its methods, handling events, and managing special elements is key to effective web automation. This section breaks down these important aspects.
WebDriver Methods and Properties
Selenium WebDriver offers various methods and properties that allow users to interact with web elements. Key methods include find_element
and find_elements
, which help locate elements on the page.
For example, driver.get("URL")
navigates to a web page. WebDriver also supports useful properties, such as current_url
to get the current page URL, and title
for the page’s title.
A common approach is to use locators like ID, name, XPath, or CSS selectors. Utilizing these effectively helps streamline automation tasks.
Event-Driven Web Automation
Event-driven automation involves responding to user interactions and web events. Selenium can trigger actions based on events like clicks or input fields.
For instance, to click a button, one might use element.click()
. Handling JavaScript events is also possible. Users can execute scripts directly via driver.execute_script("script")
.
Monitoring browser history is another aspect of event handling. Selenium allows users to manipulate the session and manage cookies, enhancing control over web automation.
Handling Special Elements and Events
Special elements include alerts, pop-ups, and frames. Selenium WebDriver provides specific methods to deal with these challenges.
For handling alerts and pop-ups, the Alert
class is useful. Methods such as accept()
and dismiss()
help manage these interruptions.
Frames and iframes also require special handling. Users must switch to a frame using driver.switch_to.frame()
to interact with elements within it.
Incorporating special keys is sometimes necessary. Using the Keys
class allows users to simulate key presses such as Enter, Backspace, or special characters in input fields.
By mastering these areas, users can enhance their proficiency with Selenium WebDriver and create more effective automation scripts.
Enhancing Browser Interactions
Enhancing browser interactions in Selenium with Python involves managing browser windows, handling frames, and executing JavaScript. These techniques allow for more effective automation and interaction with web applications.
Managing Browser Windows and Tabs
Selenium provides a way to handle multiple browser windows and tabs. Each window or tab has a unique identifier called a window handle.
To switch between windows, the command driver.switch_to.window(window_handle)
is used. To retrieve all window handles, the driver.window_handles
command returns a list of them. This feature is useful when dealing with pop-ups or multiple tabs and ensures the script interacts with the correct window.
Example:
original_window = driver.current_window_handle
for handle in driver.window_handles:
if handle != original_window:
driver.switch_to.window(handle)
This code snippet switches focus to a new window while keeping track of the original.
Handling Frames and Nested Elements
Frames and iframes can complicate browser interactions since elements within them are isolated from the main document. To interact with elements inside a frame, one must switch context using driver.switch_to.frame(frame_reference)
.
A frame reference can be an index, a name, or a WebElement object. Once inside the frame, standard Selenium commands will work for interacting with elements. After completing the tasks, returning to the main document is crucial and can be done with driver.switch_to.default_content()
.
Example:
driver.switch_to.frame('frame_name')
element = driver.find_element_by_css_selector('selector')
This allows interaction with an element within the specified frame.
Javascript Execution and Interactions
Executing JavaScript allows for more advanced control over the browser’s behavior. Selenium provides the execute_script
method, enabling users to run JavaScript code directly.
This can be particularly useful for dynamic elements where standard Selenium commands may fail. Users can manipulate the DOM, retrieve data, or even create new elements on the fly.
Example:
driver.execute_script("alert('Hello, World!');")
This line creates an alert box in the browser. JavaScript also assists in handling CSS properties if traditional methods are insufficient.
With these techniques, users can significantly enhance their browser interactions while automating tasks with Selenium and Python.
Synchronization Strategies
Achieving reliable execution in test automation requires careful handling of timing issues. Effective synchronization strategies are vital for ensuring that web elements are ready for interaction before executing actions in Selenium scripts. This section explores key techniques, such as explicit and implicit waits, and highlights fluent waits and expected conditions.
Explicit and Implicit Waits
Explicit Waits are set for specific conditions to occur before proceeding. A Selenium script will pause and check for a certain condition, such as an element being clickable. The wait time can be customized, providing precision for the required action. This ensures that tests run successfully without causing unnecessary errors.
In contrast, Implicit Waits apply a general wait time for all elements in the script. When an element is not immediately present, Selenium will keep checking until the specified time elapses. While this strategy is simpler, it can lead to longer wait times for all elements, even when some are available sooner.
Choosing between these two methods can impact the efficiency and speed of test executions. For more on different wait strategies, explore Playwright vs Selenium.
Fluent Waits and Expected Conditions
Fluent Waits combine elements of both explicit waits and the ability to ignore specific exceptions. They allow more dynamic control over waiting conditions. This is useful in scenarios where elements may change rapidly. By defining a polling interval, the script can check for conditions at regular intervals while avoiding unnecessary delays.
Expected Conditions enhance the power of fluent waits by specifying the precise condition to wait for, such as visibility or clickability of an element. This targeted approach minimizes waiting time and improves the reliability of the test. Together, these strategies allow for more adaptable tests that handle dynamic web applications effectively.
Testing and Framework Integration
Effective testing is crucial in software development, especially when using tools like Selenium with Python. This section covers how to implement unit testing and integrate frameworks like PyTest, allowing for efficient test case management and reporting.
Unit Testing with Selenium
Unit testing in Selenium is vital for ensuring that individual components of a web application function correctly. Utilizing the built-in unittest
framework, test scripts can be created to automate interactions with web elements.
Developers write test cases that include setup and teardown methods to prepare the environment and clean up afterward. A sample test case might look like this:
import unittest
from selenium import webdriver
class TestExample(unittest.TestCase):
def setUp(self):
self.driver = webdriver.Chrome()
def test_title(self):
self.driver.get("http://example.com")
self.assertEqual(self.driver.title, "Example Domain")
def tearDown(self):
self.driver.quit()
This structure helps in validating functionalities while maintaining a clean testing environment.
Integration with PyTest
Integrating Selenium with PyTest enhances testing capabilities. PyTest provides an easier syntax and powerful functionalities like fixtures and parameterization, which allow for more readable and efficient test scripts.
For example, using fixtures, a developer can create a setup method that prepares the browser instance:
import pytest
from selenium import webdriver
@pytest.fixture
def browser():
driver = webdriver.Chrome()
yield driver
driver.quit()
def test_example(browser):
browser.get("http://example.com")
assert browser.title == "Example Domain"
This code structure promotes clarity and reusability in test scripts. With PyTest, running and organizing test cases becomes more streamlined.
Building Test Suites and Reporting
Test suites can be built to run multiple test cases together, making it easier to handle large projects. Using unittest
, developers can group tests and generate reports.
To create a test suite, use the following approach:
def suite():
suite = unittest.TestSuite()
suite.addTest(TestExample('test_title'))
return suite
if __name__ == "__main__":
runner = unittest.TextTestRunner()
runner.run(suite())
Additionally, using tools like Selenium Test Automation helps in boosting testing efficiency by providing comprehensive reporting options. Detailed reports assist teams in tracking test outcomes, asserting that all features function as expected.
Advanced Selenium Techniques
This section covers two advanced techniques that enhance the effectiveness of Selenium with Python. These approaches improve code organization and optimize test execution across different environments.
Page Object Model Implementation
The Page Object Model (POM) is a design pattern used to enhance test code organization and maintainability. With POM, each webpage is represented as a class, containing methods that define interactions with its elements. This separation allows for easier updates to test code when the UI changes.
Key benefits of using POM include:
- Improved Readability: Each page class encapsulates its behavior, making tests easier to read and understand.
- Reduced Code Duplication: Common actions can be reused across tests, minimizing maintenance effort.
Developers can implement this by creating a base class that initializes the WebDriver. Then, specific page classes can inherit from it. They can define locators and actions, enhancing collaboration and community support. Many developers share POM implementations as open-source frameworks on platforms like GitHub.
Enhanced Browser Automation with Grid
Using Selenium Grid allows for distributed testing across multiple machines and browsers. This setup improves test execution speed and provides broader testing coverage. By employing a Remote WebDriver, tests can run on different environments without changing the test code.
Key aspects of Selenium Grid include:
- Parallel Testing: Multiple tests can run simultaneously, significantly reducing the total testing time.
- Cross-Browser Testing: Tests can be executed on various browser versions and platforms, increasing reliability.
To set up Selenium Grid, one can follow a comprehensive guide that explains configuration steps for effective test automation. It enables coordination between multiple nodes, allowing for a flexible, scalable testing approach. For detailed setup instructions, refer to the tutorial on Selenium Grid setup.
Final Touches and Best Practices
Attention to detail is essential for successful Selenium projects. Various best practices can improve maintainability and performance for better automation experiences. Here are important aspects to consider.
Capturing Screenshots and Videos
Capturing screenshots during test execution can be invaluable for diagnosing issues. Selenium provides a straightforward way to take screenshots with the get_screenshot_as_file()
method, which saves the image in the desired location. Including timestamps in screenshot names can help track when the tests were run.
For more detailed debugging, consider recording video of the test runs. Tools like Selenium Grid can easily integrate with screen recording software to capture the entire browser session. This allows developers to see not only the state of the application but also how users interact with it.
Cookies and Sessions Management
Managing cookies and sessions in Selenium is crucial to maintain the state of the application. Selenium allows users to add, delete, and modify cookies using methods like add_cookie()
, delete_cookie()
, and get_cookies()
. This functionality ensures that sessions remain active between test runs.
Additionally, developers should be aware of session management practices. For automated tests that require login, storing authentication cookies can save time and resources. Always ensure to delete cookies at the end of the session to prevent conflicts during future test executions.
Optimizing Test Performance
Test performance can be a deciding factor for smooth automation. To optimize, utilize techniques such as parallel execution and test case prioritization. Running tests concurrently on multiple browsers or using Selenium Grid can significantly reduce execution time.
Furthermore, avoid using unnecessary waits. Instead of using explicit waits, developers should focus on implicit waits, which can improve performance by waiting just the right amount for elements to load. Regularly reviewing and refining test cases can also contribute to better performance and faster feedback loops.
Code Maintenance and Documentation
Good documentation and code maintenance practices are essential for sustainable automation. Keeping code organized and using the Page Object Model can aid maintainability. This design pattern separates test logic from UI interactions, making code easier to manage and update.
Using platforms like GitHub for version control ensures that changes are tracked and collaborative efforts are simplified. Writing clear and concise comments within the code helps other developers understand the logic behind test cases, reducing onboarding time for new team members.
Regularly reviewing and refactoring code ensures that tests remain relevant and efficient. This dedication to maintainability benefits both current and future automation efforts.
Frequently Asked Questions
This section addresses common inquiries regarding Selenium with Python. It covers setup, tutorials, best practices, handling elements, differences between tools, and integration with continuous systems. Each question targets essential aspects that contribute to effective automation testing.
How can I set up Selenium with Python for automation testing?
To set up Selenium with Python, one must first install the Selenium package using pip. A typical command is pip install selenium
. Next, download a compatible WebDriver for the browser being used, such as ChromeDriver for Google Chrome, and ensure it is accessible in the system’s PATH.
Where can I find good tutorials and examples for Selenium with Python?
There are various online resources available for learning Selenium with Python. Websites and blogs focusing on programming, such as tutorials on Selenium Interview Questions: Insights from Industry Experts, offer comprehensive guides and practical examples that enhance understanding and skills.
What are best practices for using Selenium with Python for web scraping?
When using Selenium for web scraping, it is recommended to manage browser windows efficiently. Using implicit and explicit waits can prevent issues with slow-loading elements. Additionally, employing try-except blocks helps to handle exceptions gracefully, allowing scripts to run without interruption.
How do I handle elements in Selenium using Python?
Elements in Selenium can be handled using various locating strategies such as ID, XPath, or CSS selectors. Once located, methods like .click()
or .send_keys()
can be used to interact with these elements. It is crucial to ensure the target elements are loaded before operations to avoid errors.
What are the differences between Selenium WebDriver and Selenium RC in the context of Python?
Selenium WebDriver is the current standard and is more powerful than Selenium RC. WebDriver directly controls the browser by communicating with its native components, while RC requires a server to interpret commands. This makes WebDriver faster and more reliable for automation tasks.
Can you explain how to integrate Selenium with Python for continuous integration systems?
Integration of Selenium with Python in continuous integration systems can be achieved by using testing frameworks like pytest or unittest. These frameworks allow the creation of automated test scripts that can be executed in CI/CD pipelines, ensuring code quality and functionality across various stages of development.