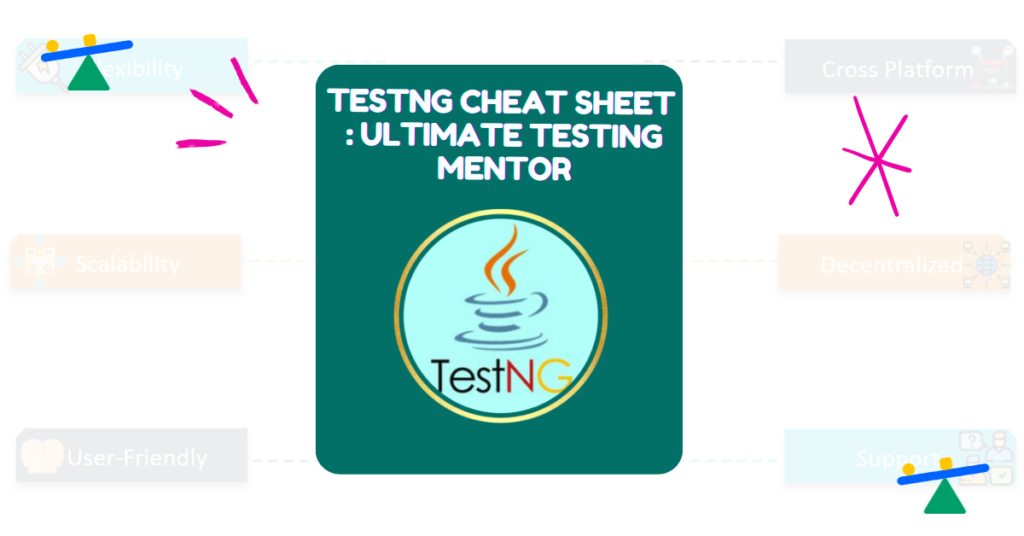
In the world of software testing, TestNG stands out as a powerful testing framework inspired by JUnit and NUnit. It is designed to cover all categories of tests: unit, functional, end-to-end, and integration. This blog post will provide you with a comprehensive TestNG cheat sheet, helping you navigate its features and functionalities with ease.
To make this resource even more valuable, we are offering a PDF version of this cheat sheet. Simply subscribe using the subscription box on the right side or leave your email address in the comments below, and we will send it to you right away! Below is the PNG Image of same:
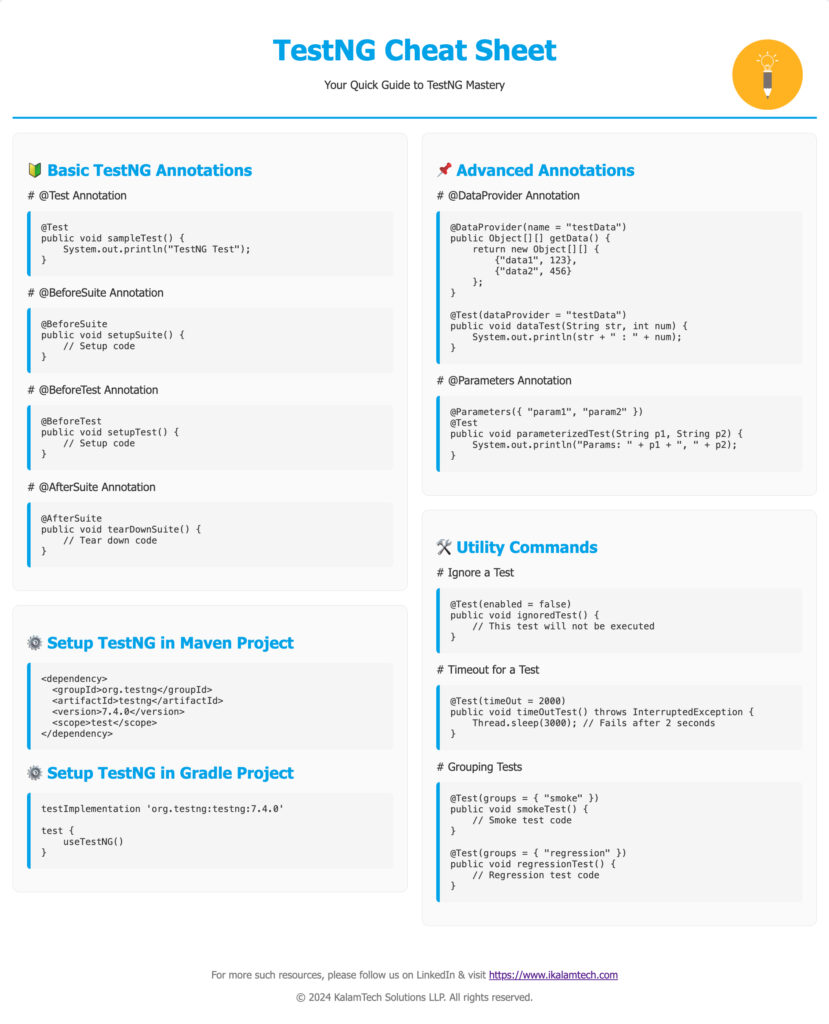
What is TestNG?
TestNG (Test Next Generation) is an open-source testing framework that simplifies the process of writing and running tests. It is particularly useful for Java developers and testers. TestNG provides advanced features such as:
- Annotations: Simplifies test configuration.
- Flexible Test Configuration: Allows grouping of tests in various ways.
- Data-Driven Testing: Supports parameterization of tests.
- Parallel Execution: Facilitates running tests concurrently to save time.
Key Features of TestNG
1. Annotations
TestNG uses annotations to define test methods and configure test execution. Here are some of the most commonly used annotations:
@Test
: Marks a method as a test method.@BeforeSuite
: Executed before the suite starts.@AfterSuite
: Executed after the suite ends.@BeforeClass
: Executed before the first method of the current class is invoked.@AfterClass
: Executed after all the methods of the current class are invoked.@BeforeMethod
: Executed before each test method.@AfterMethod
: Executed after each test method.@DataProvider
: Provides data to test methods.
2. Test Configuration
TestNG allows you to configure your tests flexibly. You can group tests, run tests in a specific order, and even skip tests based on conditions. Here’s how you can configure tests:
- Grouping Tests: You can group tests using the
groups
attribute in the@Test
annotation.
@Test(groups = {"smoke"})
public void smokeTest() {
// test code
}
- Dependency Testing: You can specify dependencies between tests using the
dependsOnMethods
attribute.
@Test(dependsOnMethods = {"testMethod1"})
public void testMethod2() {
// test code
}
3. Data-Driven Testing
TestNG supports data-driven testing through the @DataProvider
annotation. This allows you to run the same test with different sets of data.
@DataProvider(name = "data-provider")
public Object[][] dataProviderMethod() {
return new Object[][] {
{ "data1" },
{ "data2" }
};
}
@Test(dataProvider = "data-provider")
public void testMethod(String data) {
// test code using data
}
4. Parallel Execution
TestNG allows you to run tests in parallel, which can significantly reduce execution time. You can configure parallel execution in the TestNG XML file:
<suite name="Suite" parallel="methods" thread-count="5">
<test name="Test1">
<classes>
<class name="com.example.TestClass1"/>
</classes>
</test>
<test name="Test2">
<classes>
<class name="com.example.TestClass2"/>
</classes>
</test>
</suite>
5. Reporting
TestNG generates detailed reports after test execution. You can view the results in the console or generate HTML and XML reports. This feature helps in tracking the status of your tests and identifying any failures.
TestNG XML Configuration
TestNG uses an XML file to configure the test suite. Here’s a simple example of a TestNG XML configuration:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="Suite">
<test name="Test">
<classes>
<class name="com.example.TestClass"/>
</classes>
</test>
</suite>
How to Run TestNG Tests
You can run TestNG tests in various ways:
- Using an IDE: Most IDEs like IntelliJ IDEA and Eclipse support TestNG. You can run tests directly from the IDE.
- Command Line: You can run TestNG tests using Maven or Gradle.
- Using XML: Run tests defined in a TestNG XML file via the command line.
Best Practices for Using TestNG
- Keep Tests Independent: Ensure that tests do not depend on each other to avoid cascading failures.
- Use Descriptive Names: Name your test methods descriptively for better readability and maintenance.
- Organize Tests: Group related tests together to maintain clarity and structure.
- Leverage Data Providers: Use data providers for data-driven testing to enhance test coverage without duplicating code.
- Implement Logging: Use logging to track test execution and debug failures effectively.
Integrating TestNG with Selenium
TestNG can be extremely valuable when combined with the Selenium automation tool. Selenium is widely used for automating web applications, and TestNG enhances its capabilities by providing structured test management, reporting, and parallel execution.
Benefits of Using TestNG with Selenium
- Improved Test Management: TestNG’s annotations and XML configuration allow for better organization of Selenium tests.
- Parallel Execution: You can run multiple Selenium tests in parallel, significantly reducing the time needed for regression testing.
- Data-Driven Testing: Use TestNG’s data providers to run Selenium tests with multiple sets of input data, ensuring comprehensive test coverage.
- Detailed Reporting: TestNG provides detailed reports that help in analyzing test results and identifying issues.
If you are looking to dive deeper into Selenium and enhance your automation skills, consider enrolling in our comprehensive online course. This course covers everything you need to know to get started with Selenium, including:
- In-depth explanations of Selenium features
- Practical examples and exercises
- Advanced techniques and best practices
You can find the course here: Selenium Automation Course.
Conclusion
TestNG is a powerful testing framework that, when combined with Selenium, can significantly enhance your automation testing efforts. This cheat sheet provides a quick reference to the essential features and functionalities of TestNG, helping you streamline your testing processes.
For a PDF version of this cheat sheet, remember to subscribe using the box on the right side or leave your email in the comments below. Happy testing!