Selenium WebDriver is a powerful tool that allows developers to automate web applications for testing purposes. Certainly, with this tutorial, readers will gain the confidence to set up and use Selenium WebDriver effectively, enabling them to create reliable automated tests. Additionally, this comprehensive guide will also cover the essential features of Selenium, including how to interact with web pages and integrate testing frameworks seamlessly.

By understanding the core components and advanced features of Selenium WebDriver, developers can enhance their testing strategies. They will learn best practices and design patterns that promote clean and maintainable code. This tutorial aims to provide a clear roadmap for beginners and experienced users alike, ensuring that they feel knowledgeable about the latest updates in Selenium.
In addition, the article will touch upon cross-browser testing and practical examples that demonstrate the capabilities of Selenium WebDriver. Readers will come away with the skills needed to start developing their own automated testing projects confidently.
Key Takeaways
- Readers will learn how to set up and use Selenium WebDriver effectively.
- The tutorial covers advanced features and best practices for automation testing.
- Practical examples will help readers develop their own testing projects confidently.
Getting Started with Selenium WebDriver
Understanding selenium WebDriver & its components and setting up the environment correctly is crucial for effective testing.
Overview of Selenium Tools
Selenium is an open-source suite that comprises several tools for web automation. Key components include:
- Selenium IDE: A user-friendly tool for creating quick test scripts without programming.
- Selenium RC: The original version that allows testers to write tests in various programming languages, now largely replaced by WebDriver.
- Selenium Grid: Enables parallel execution of tests across different machines and browsers.
These tools cater to different needs in the testing process. Selenium WebDriver is most favored for its direct interaction with browsers, making it essential for modern automation.
Setting Up the Selenium Environment
To get started with Selenium WebDriver, the following steps are essential:
- Install Java JDK: Selenium requires Java to run. Download and install the latest version from the official website.
- Download Selenium WebDriver: Get the latest Selenium WebDriver from the Selenium website.
- Set Up Browser Drivers: Each browser requires a specific driver (for example, ChromeDriver for Google Chrome). Download the driver version compatible with the browser.
- Configure the IDE: Set up an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA, including the necessary libraries.
Ensuring all components are compatible and properly set up is crucial for seamless automation.
Understanding WebDriver and Browsers
WebDriver acts as an interface between the test script and the browser. It controls the browser by sending commands for actions like clicking buttons and filling forms.
Selenium WebDriver supports multiple browsers, including:
- Google Chrome
- Mozilla Firefox
- Safari
- Microsoft Edge
Each browser has its specific driver that translates the WebDriver commands into browser-specific actions. For example, Chrome uses ChromeDriver, while Firefox uses GeckoDriver. This diversity allows testers to run their scripts on various platforms and operating systems, enhancing flexibility and coverage in testing.
Core Components of Selenium WebDriver
Selenium consists of several key components that facilitate automated web testing. Understanding these components is essential for effective use of Selenium WebDriver in various programming environments.
WebDriver API Explained
Selenium WebDriver API serves as the core interface for browser automation. It provides simple and concise commands for interacting with web elements. The API allows automation testers to perform actions like clicking buttons, entering text, and navigating between pages.
WebDriver is designed to support multiple programming languages through language bindings. This flexibility enables users to write test scripts in languages such as Java, C#, Python, and Ruby. The API uses browser-specific drivers to communicate with the browser, ensuring consistent behavior across different web platforms.
Selenium Client Libraries
Selenium Client Libraries are essential for linking the WebDriver API with the chosen programming language. These libraries provide the necessary functions and classes that facilitate communication with the WebDriver.
For instance, the Java client library includes classes like WebDriver
, WebElement
, and various exception handling tools. Each client library has its unique features and functionalities, aligning with the syntax and structure of the respective languages.
To set up the libraries, one typically needs to include the library jar files in their project or install them via package managers like Maven or npm. This setup is crucial for seamless integration and testing automation.
Browser Automation Commands
Browser automation commands are specific actions that can be executed on web elements. Fundamental commands include clicking, sending keys, and retrieving element attributes. These commands allow testers to interact with dynamic web applications accurately.
Each command corresponds to methods in the WebDriver API. For example, the click()
method initiates a click action, while sendKeys()
inputs text into a field.
Additionally, commands can be combined to create complex scenarios, such as filling out forms or navigating through multi-step processes. This flexibility makes Selenium a powerful tool for comprehensive web testing automation.
Interacting with Web Pages
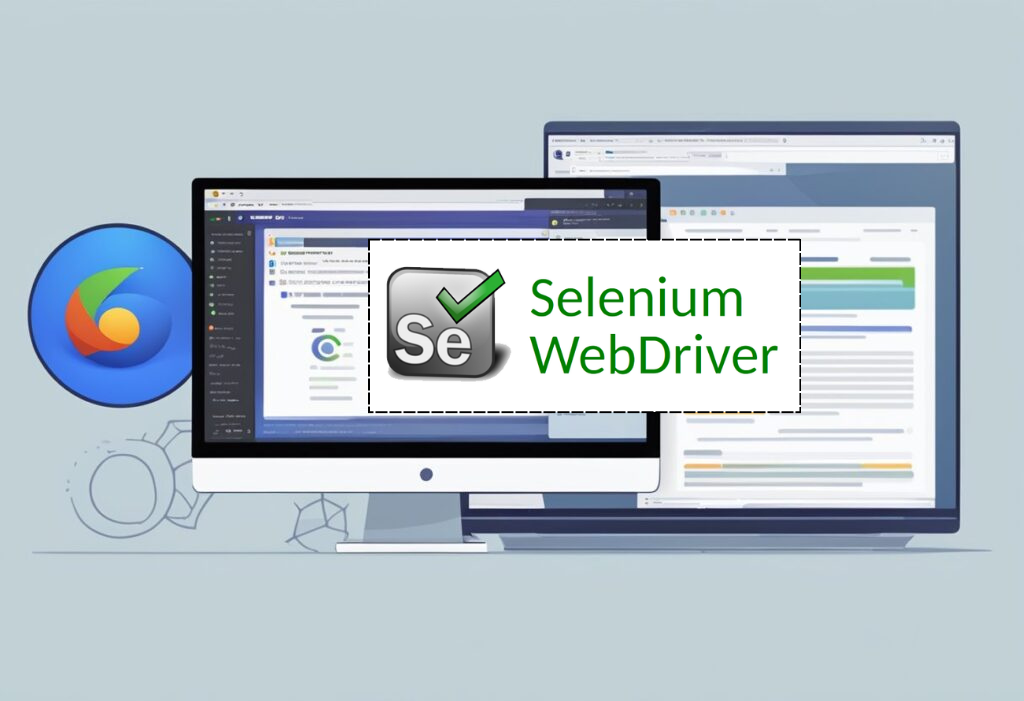
Interacting with web pages is a critical skill when using Selenium WebDriver. This includes identifying web elements, using commands to manipulate those elements, and handling more complex features of web applications. Understanding these aspects enhances the automation process significantly.
Identifying Web Elements
To interact effectively with web pages, one must identify web elements accurately. Selenium provides various methods for locating elements, including ID, name, class name, CSS selectors, and XPath.
CSS Selectors allow users to select elements based on styling attributes. For example, driver.findElement(By.cssSelector(".className"))
retrieves elements with a specific class.
XPath is another powerful tool that navigates the DOM structure. An example is driver.findElement(By.xpath("//div[@id='example']"))
, which targets a <div>
with a specific ID.
Most commonly used for identifying elements, these methods significantly streamline the interaction process.
WebElement Commands
Once identified, web elements can be manipulated using various WebElement commands. These commands include actions like click, sendKeys, and getText.
The click()
method is essential for simulating user clicks. For instance, element.click()
triggers a click event.
For entering text into input fields, sendKeys("text")
is used. For example, element.sendKeys("Hello World")
enters “Hello World” into a text box.
To retrieve text, getText()
provides the visible text of an element. By mastering these commands, one can simulate a variety of user interactions.
Handling Complex Page Features
In modern web applications, handling complex features such as iframes and mouse hover actions is essential. Iframes are used to embed content from another source. To switch to an iframe, driver.switchTo().frame("frameName")
is used.
For mouse hover actions, Selenium requires JavaScriptExecutor to perform tasks that are not directly supported. For instance, executing a JavaScript snippet to hover over an element can be accomplished with ((JavascriptExecutor) driver).executeScript("arguments[0].dispatchEvent(new MouseEvent('mouseover'));", element);
.
By mastering these techniques, users can navigate even the most complex web applications efficiently.
Advanced WebDriver Features
Advanced features in Selenium WebDriver enable users to manage browser behavior, interact with web elements programmatically, and handle security settings. These capabilities enhance the testing experience and allow for greater control over the automation process.
Managing Browser Windows and Alerts
Selenium WebDriver allows the management of multiple browser windows and alerts effectively. It can switch between windows using the command driver.switchTo().window(windowHandle)
, where windowHandle
is the unique ID of the window. This is crucial for scenarios where multiple pop-ups or tabs are opened during testing.
Handling alerts is straightforward. With driver.switchTo().alert()
, users can interact with JavaScript alerts, confirm dialogs, and prompts. For example, to accept an alert, the command is simply alert.accept()
. Similarly, alert.dismiss()
can cancel an alert or confirmation dialog. This functionality ensures that automation scripts can proceed seamlessly, even with interruptions from alerts.
Scripting with JavaScriptExecutor
The JavaScriptExecutor interface in Selenium allows the execution of JavaScript code within the context of the loaded page. This is valuable when standard WebDriver methods are insufficient.
To use JavaScriptExecutor, first, cast the WebDriver instance:
JavascriptExecutor js = (JavascriptExecutor) driver;
With this, users can run scripts like js.executeScript("return document.title;")
to retrieve the page title. It can also be used to manipulate page elements, such as scrolling to a certain part of the page or changing element styles. This feature gives testers the flexibility to achieve actions that are not possible through standard commands, enhancing their testing strategies.
Handling Cookies and SSL Certificates
Cookies play an essential role in web applications, and Selenium makes it easy to manage them. Users can add, delete, and retrieve cookies using commands like driver.manage().addCookie(cookie)
and driver.manage().deleteCookieNamed(name)
.
For handling SSL certificates, WebDriver simplifies the process. By setting desired capabilities, users can bypass SSL certificate errors. For example, in Chrome, they can include this in their setup:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("acceptInsecureCerts", true);
This allows the automation process to run smoothly even on sites with untrusted certificates, ensuring tests do not fail due to security warnings.
Test Frameworks Integration
Integrating Selenium with a testing framework enhances automation testing efficiency. This section focuses on how to use Selenium with TestNG, create a Selenium Test Suite, and implement parallel testing.
Using Selenium with TestNG
TestNG is a popular choice for integrating with Selenium due to its powerful features. It allows for easy management of test cases through XML configuration files. TestNG annotations like @Test
, @BeforeClass
, and @AfterClass
help organize tests effectively.
This framework also supports data-driven testing using the @DataProvider
annotation. By feeding multiple sets of data into a single test method, developers can test various scenarios efficiently. Additionally, TestNG reports help monitor test execution, making it easier to identify issues rapidly.
Creating Selenium Test Suite
A Selenium Test Suite lets testers group multiple test cases for systematic execution. TestNG simplifies this process through its XML suite configuration file, which specifies test classes, methods, and desired configuration settings.
Testers can define multiple suites within a single XML file, allowing for various testing requirements. This setup is beneficial for large projects, as it helps maintain organization and clarity. The inclusion of <suite>
, <test>
, and <classes>
tags aids in structuring test cases in a clear hierarchy.
Parallel Testing in Selenium
Running tests in parallel significantly speeds up the testing process. This is crucial for large applications where execution time can become a bottleneck.
TestNG supports parallel execution by setting the parallel
attribute in the suite XML file. Users can specify whether tests, classes, or methods should run in parallel. It’s essential to ensure that tests do not interfere with each other, especially when they share resources.
Additionally, using programming languages like Java, C#, Python, or Ruby enables flexibility in how these tests are written and executed. Proper configuration ensures maximum efficiency during the testing process.
Design Patterns and Best Practices
In the world of Selenium WebDriver, using design patterns and best practices is essential for creating effective and maintainable test scripts. Key concepts include the Page Object Model, the Factory Pattern with PageFactory, and strategies for maintaining clean code.
Page Object Model
The Page Object Model (POM) is a widely-used design pattern in test automation where it helps to separate test scripts from the UI elements. Each web page is represented by a class, where elements are defined using locators. This approach enhances code maintainability.
With POM, if a page structure changes, only the page object class needs updating. The tests can remain untouched. This minimizes maintenance effort and promotes reuse of code across different tests, making it integral to automated frameworks.
Factory Pattern with PageFactory
The Factory Pattern, combined with PageFactory, simplifies object creation for page models. Instead of initializing elements manually in the constructor, PageFactory automates this process. Developers can use the @FindBy
annotation, making it easier to manage and locate web elements.
This pattern reduces clutter in the code and improves readability. With PageFactory, when a test is run, the Selenium framework dynamically initializes page objects. This keeps the test code organized and fosters better practices for creating maintainable test scripts.
Strategies for Maintainable Test Code
Creating maintainable test scripts is crucial for long-term success in automation. Some effective strategies include using descriptive naming conventions, modular test cases, and proper commenting.
By using clear naming conventions for test methods and classes, testers can quickly understand their purpose. Hence, Modular test cases allow for easier updates and debugging. Comments should explain the intent behind complex logic.
Incorporating these methods leads to cleaner, more understandable code. This minimizes confusion for new team members and enhances the overall quality of the automation framework.
Cross-Browser and Advanced Testing
Testing across different web browsers is crucial to ensure that applications function well for all users. Selenium provides tools for effective cross-browser testing and advanced options to improve testing efficiency.
Configuring Browser Drivers for Parallel Testing
To begin parallel testing, one must first configure the appropriate browser drivers. Each browser has its own driver, such as ChromeDriver for Google Chrome and Geckodriver for Mozilla Firefox.
To set up a driver, follow these steps:
- Download the Driver: Get the correct version of the browser driver from its official source.
- Set the Driver Path: Specify the driver path in the script using
System.setProperty()
. - Initiate the Browser: Create an instance using the WebDriver interface.
Using these drivers in parallel allows multiple tests to run simultaneously. This saves time and enhances testing efficiency.
Cross-Browser Testing with Selenium Grid
Selenium Grid allows users to run tests on different browsers and operating systems concurrently. It consists of a Hub and multiple Nodes.
- Hub: This is the central point where test commands are sent.
- Nodes: Nodes are the machines where the tests are executed.
To use Selenium Grid:
- Start the Hub: Run the hub using a command line.
- Register Nodes: Connect nodes to the hub for testing.
Cross-browser testing using Selenium Grid helps ensure browser compatibility. It allows for comprehensive testing on different environments without needing multiple setups.
Headless Browser Testing
Headless browser testing lets users run tests without a graphical user interface (GUI). This is useful for quicker execution, especially for automated tests.
Common headless browsers include:
- HtmlUnit: Lightweight and designed for unit testing.
- PhantomJS: Known for its capability to handle page rendering without showing a window.
To implement headless testing in Selenium:
- Set Up Desired Capabilities: Modify the browser capabilities for headless execution.
- Instantiate the Driver: Create the WebDriver instance with headless settings.
Headless testing not only speeds up tests but also optimizes resource usage, making it ideal for continuous integration (CI) environments.
New Features in Selenium 4
Selenium 4 brings significant improvements that enhance its usability and performance. Key advancements include the adoption of the W3C Protocol, which simplifies the interaction between the driver and the browser. Additionally, upgrading from Selenium 3 to Selenium 4 offers important features that developers should know.
W3C Protocol and Selenium 4 Enhancements
One of the most important updates in Selenium 4 is the implementation of the W3C Protocol. This protocol ensures that all browser interactions are standardized, leading to fewer compatibility issues across different platforms.
With the W3C Protocol, Selenium WebDriver has improved its architecture. Communication is now more reliable and efficient as it minimizes the discrepancies that existed in earlier versions.
Developers can now use new command syntax that aligns with modern web standards. This change leads to more predictable behavior when automating browser actions.
Additionally, these enhancements make it easier to manage sessions and reduce the likelihood of errors during test execution. Consequently, testers experience smoother automated workflows.
Upgrading from Selenium 3 to Selenium 4
Transitioning from Selenium 3 to Selenium 4 involves some notable changes. First, users should be aware of the updated API. Many commands have been revised to conform with the new standards.
For instance, deprecated commands from Selenium 3 have been removed. Maintaining legacy code may require adjustments to ensure compatibility with the new framework.
Furthermore, Selenium 4 offers improved support for modern web features. Features like relative locators make it easier to identify web elements in relation to others.
Documentation is also more comprehensive, offering resources that guide new users through the upgraded system. This support simplifies the learning curve for those familiar with Selenium 3.
By understanding these updates and the overall architecture, developers can leverage Selenium 4’s capabilities to enhance their automated testing efforts.
Developing a Sample WebDriver Project
Creating a sample WebDriver project involves careful planning and writing effective test scripts. This process is essential for anyone looking to understand automation testing using Selenium WebDriver.
Planning and Structuring Your Test Project
Before starting, define the goals and scope of the project. Identify the application to be tested and the features that require testing. Consider using a Selenium WebDriver Framework to organize and manage test cases efficiently.
Establish a folder structure for the Selenium Project. A typical structure may include:
- src: Contains test scripts and resources.
- lib: Holds necessary libraries and dependencies.
- reports: Stores test execution reports.
Using a clear structure will help maintain organization as the project expands. So, Create a test plan to outline the test cases, expected results, and any specific tools required. This structured approach aids in executing automation testing smoothly.
Writing and Executing a Test Script
When writing a Selenium WebDriver Script, start by setting up the environment and ensure that the necessary software is installed, including the appropriate WebDriver executable for the browser being tested.
Write a simple test script to navigate to a web page, such as opening Google. Here’s a basic outline:
- Import the required libraries.
- Initialize the WebDriver.
- Use commands to navigate and interact with web elements.
For example:
WebDriver driver = new ChromeDriver();
driver.get("http://www.google.com");
Executing the script will launch the browser and navigate to the specified URL. Thereafter, Monitor the output for errors and troubleshoot as necessary. Automating test scripts streamlines the testing process and enhances efficiency in software development.
Learning Selenium Advanced: Resources and Recommendations
If you’re serious about mastering Selenium, several resources can help you build your skills systematically:
1. Selenium Cheat Sheets
Download our Selenium cheat sheets in different programming languages:
- Java Selenium Cheat Sheet (PDF)
- Python Selenium Cheat Sheet (PDF)
- JavaScript Selenium Cheat Sheet (PDF)
2. Online Courses
There is a comprehensive online course that covers all the topics you need to get a head start with Selenium. Not only this course is perfect for beginners looking to start their automation journey but also for those already in the field who wish to upskill.
The course includes:
- In-depth explanations of Selenium features
- Practical examples and exercises
- Advanced techniques and best practices
You can find the course here.
Make sure to leverage the resources we’ve provided, and don’t hesitate to reach out for more insights and assistance in your automation journey!